This is a brief tutorial describing how to create a Web service using WSDL and annotations.
The approach presented here allows you to design and implement your WSDL/Schema and Java classes
independently without having to generate anything. You can then use annotations
to map Java classes to appropriate WSDL and Schema elements.
Since JAXB and JAX-WS have sensible defaults, the number of annotations can be kept
to the minimum.
In my opinion, it is always best to develop WSDL and schemas by hand to ensure that the
service contract is appropriately defined and also that the schema
can be re-used (by other services) and extended if necessary. I do not recommend using annotations
for automatically producing WSDL and schemas at runtime as this leads to
simplistic schemas and WSDLs.
Generating classes from WSDL/schema sometimes makes sense, say in situations
when you have to use a pre-defined schema. However, as with any code generation,
it creates a maitainance problem especially if there is a need to put behavior
into generated classes. So it is desirable to be able to evolve object model and
service implementation classes independently
from WSDL/schemas. You can do it quite easily by following the steps below.
Even though I use the word “create” when describing some of the steps, the same approach
will also work for updates or refactoring caused by changes in
service requirements.
The steps are the following:
-
Design your service interface. In our case the service has a single “add” operation that accepts
a “person” document, adds it to a database and returns back the status:
public String add( Person person )
-
Design your data/object model. In our case Person class has three fields
(ssn, firstName, lastName) and the list of addresses.
Real world examples will certainly be more complex, but this is good enough for our purposes.
-
Define XML schema based on your object model.
Use strict datatypes where possible. For example, we use
xsd:token
instead of xsd:string
since “token” does not allow
carriage return, tabs, and leading spaces:
<xsd:element name="person">
<xsd:complexType >
<xsd:sequence>
<xsd:element name="ssn" type="xsd:token"
minOccurs="1" maxOccurs="1"/>
<xsd:element name="firstName" type="xsd:token"
minOccurs="1" maxOccurs="1"/>
<xsd:element name="lastName" type="xsd:token"
minOccurs="1" maxOccurs="1" />
<xsd:element name="address" type="Address"
minOccurs="1" maxOccurs="unbounded"/>
</xsd:sequence>
</xsd:complexType>
</xsd:element>
Complete schema file
Create an XML file for your schema so you can test the schema.
You can generate an XML instance document
automatically from Eclipse (assuming you’re using
Eclipse Web Tool Platform)
by right-clicking on the schema in navigator and selecting “generate”.
Test the schema by changing data and making sure that you’re getting correct
validation errors.
Sample XML file
Create WSDL. Our WSDL imports the schema that we just created.
Note that the WSDL target namespace is different from the schema namespace since the
same schema can be reused by different WSDLs:
<wsdl:definitions
targetNamespace="http://personservice"
xmlns="http://personservice"
xmlns:pers="http://person"
xmlns:soapenc="http://schemas.xmlsoap.org/soap/encoding/"
xmlns:wsdl="http://schemas.xmlsoap.org/wsdl/"
xmlns:wsdlsoap="http://schemas.xmlsoap.org/wsdl/soap/"
xmlns:xsd="http://www.w3.org/2001/XMLSchema">
<wsdl:types>
<xsd:import namespace="http://person"
schemaLocation="person.xsd" />
<!-- Return value -->
<xsd:element name="status" type="xsd:string" />
</wsdl:types>
...
Complete WSDL file
Create your object model classes and annotate them with appropriate JAXB annotations:
// By default JAXB uses getters/setters for marshaling/unmarshaling
// Using fields access allows us not to define getters/setters for
// all mapped instance variables.
@XmlAccessorType(XmlAccessType.FIELD)
// We need to provide the namespace, otherwise it defaults to
// the package name
@XmlRootElement(namespace="http://person")
public class Person {
// we only need annotations for variables that don't match
// element names
private String ssn;
private String firstName;
private String lastName;
@XmlElement(name = "address")
protected List<Address> addresses;
...
Person class
Address class
Create ObjectFactory class. This class is required by JAXB and it
should contain factory methods for all JAXB-mapped classes. ObjectFactory
must resides in the same package with object model classes.
@XmlRegistry
public class ObjectFactory {
public Person createPerson() {
return new Person();
}
public Address createAddress() {
return new Address();
}
}
ObjectFactory class
Create Web Service implementation class. Note that with JAX-WS
Service Endpoint Interface (SEI) is optional, so all you need is an implementation class:
@WebService(
// all values must match corresponding attributes
// and elements of the WSDL file
// Name of the port type in WSDL
name="PersonService",
// Target namespace of WSDL, could be
// different from the schema namespace
targetNamespace="http://personservice",
serviceName="PersonServicePorts",
portName="PersonService",
// the file must be available to Web container
wsdlLocation="WEB-INF/wsdl/PersonService.wsdl"
)
/*
* We'are using "bare" style since "add" operation
* takes only one argument, person document. Therefore, there
* is no need to "wrap" it by nesting it inside operation element.
* Note that "wrapped" style is the default.
*/
@SOAPBinding(parameterStyle=SOAPBinding.ParameterStyle.BARE)
public class PersonService {
@WebResult(name = "status")
public String add( Person person ) {
System.out.println("Adding person :"+person );
return "Added "+person.getFullName();
}
}
PersonService class
Deploy your Web service to your application server or Web services container.
Refer to your application server documentation for details.
Test the service.
The easiest way to test the service is to use open source soapUI tool.
You can install it as Eclipse plugin (there are also plugins for
NetBeans and IDEA) following these instructions.
In Eclipse, open SoapUI view, right click and create a project from your WSDL file. SoapUI will automatically
create a sample SOAP request which you can update with your data. You can run it right away.
Later, you can create a repeatable test suite with parametirized data using SoapUI properties and
assertions:
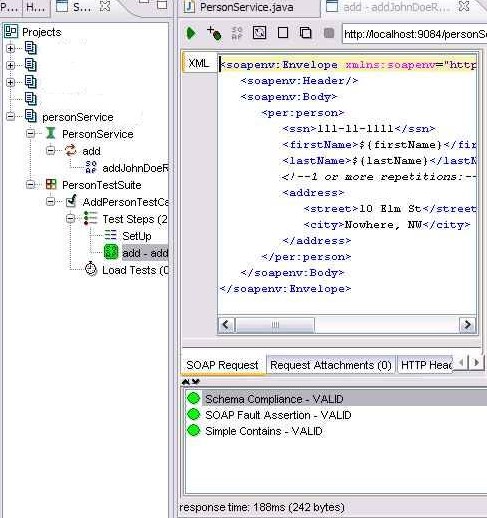
SoapUI Project (you can import it into SoapUI).
You can also download all files in a zip file.